Center Align Elements
To horizontally center a block element (like <div>), use margin: auto;
Setting the width of the element will prevent it from stretching out to the edges of its container.
The element will then take up the specified width, and the remaining space will be split equally between the two margins:
Example
<!DOCTYPE html> <html> <head> <style> .center { margin: auto; width: 60%; border: 3px solid #73AD21; padding: 10px; } </style> </head> <body> <h2>Center Align Elements</h2> <p>To horizontally center a block element (like div), use margin: auto;</p> <div class="center"> <p>Hello World!</p> </div> </body> </html>
Result:
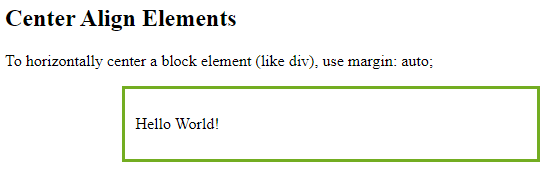
Center Align Text
To just center the text inside an element, use text-align: center;
Example
<!DOCTYPE html> <html> <head> <style> .center { text-align: center; border: 3px solid green; } </style> </head> <body> <h2>Center Text</h2> <div class="center"> <p>This text is centered.</p> </div> </body> </html>
Result:

Center an Image
To center an image, set left and right margin to auto
and make it into a block
element:
Example
<!DOCTYPE html> <html> <head> <style> img { display: block; margin-left: auto; margin-right: auto; } </style> </head> <body> <h2>Center an Image</h2> <p>To center an image, set left and right margin to auto, and make it into a block element.</p><img src="paris.jpg" alt="Paris" style="width:40%"> </body> </html>
Result:
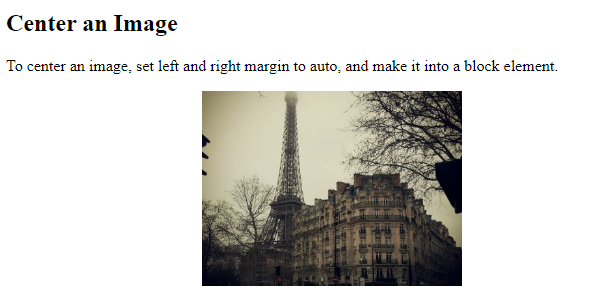
Left and Right Align – Using position
One method for aligning elements is to use position: absolute;
:
Example
<!DOCTYPE html> <html> <head> <style> .right { position: absolute; right: 0px; width: 300px; border: 3px solid #73AD21; padding: 10px; } </style> </head> <body> <h2>Right align with the position property</h2> <p>An example of how to right align elements with the position property:</p> <div class="right"> <p>In my younger and more vulnerable years my father gave me some advice that I've been turning over in my mind ever since.</p> </div> </body> </html>
Result:
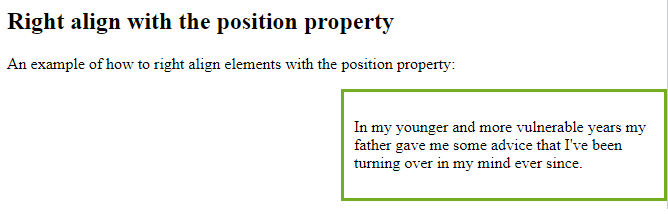
Left and Right Align – Using float
Another method for aligning elements is to use the float
property:
Example
<!DOCTYPE html> <html> <head> <style> .right { float: right; width: 300px; border: 3px solid #73AD21; padding: 10px; } </style> </head> <body> <h2>Right align with the float property</h2> <p>An example of how to right align elements with the float property:</p> <div class="right"> <p>In my younger and more vulnerable years my father gave me some advice that I've been turning over in my mind ever since.</p> </div> </body> </html>
Result:
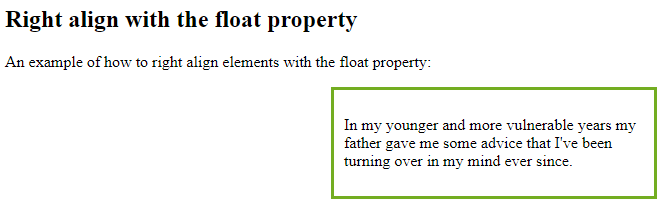
The clearfix Hack
Note: If an element is taller than the element containing it, and it is floated, it will overflow outside of its container. You can use the “clearfix hack” to fix this (see example below).
Then we can add the clearfix hack to the containing element to fix this problem:
Example
<!DOCTYPE html> <html> <head> <style> div { border: 3px solid #4CAF50; padding: 5px; } .img1 { float: right; } .img2 { float: right; } .clearfix::after { content: ""; clear: both; display: table; } </style> </head> <body> <h2>Without Clearfix</h2> <p>This image is floated to the right. It is also taller than the element containing it, so it overflows outside of its container:</p> <div> <img class="img1" src="pineapple.jpg" alt="Pineapple" width="170" height="170"> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus imperdiet... </div> <h2 style="clear:right">With New Modern Clearfix</h2> <p>Add the clearfix hack to the containing element, to fix this problem:</p> <div class="clearfix"> <img class="img2" src="pineapple.jpg" alt="Pineapple" width="170" height="170"> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus imperdiet... </div> </body> </html>
Result:
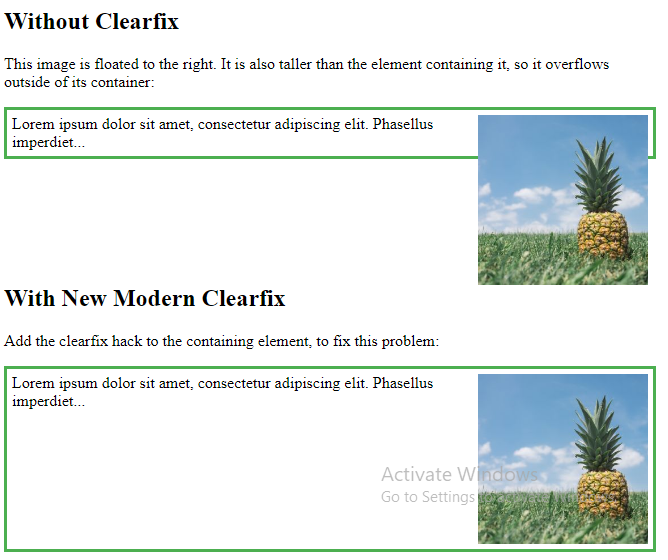
Center Vertically – Using padding
There are many ways to center an element vertically in CSS. A simple solution is to use top and bottom padding
:
Example
<!DOCTYPE html> <html> <head> <style> .center { padding: 70px 0; border: 3px solid green; } </style> </head> <body> <h2>Center vertically with padding</h2> <p>In this example, we use the padding property to center the div element vertically:</p> <div class="center"> <p>I am vertically centered.</p> </div> </body> </html>
Result:
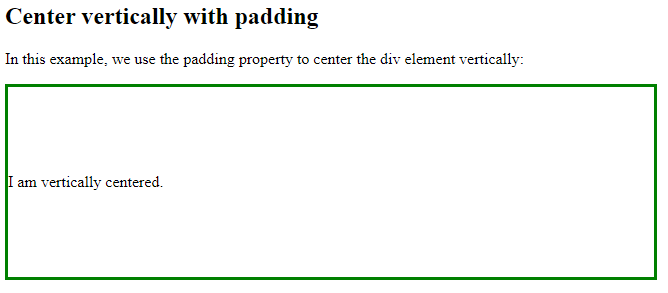
To center both vertically and horizontally, use padding
and text-align: center
:
Example
<!DOCTYPE html> <html> <head> <style> .center { padding: 70px 0; border: 3px solid green; text-align: center; } </style> </head> <body> <h2>Center with padding and text-align</h2> <p>In this example, we use padding and text-align to center the div element both vertically and horizontally:</p> <div class="center"> <p>I am vertically and horizontally centered.</p> </div> </body> </html>
Result:
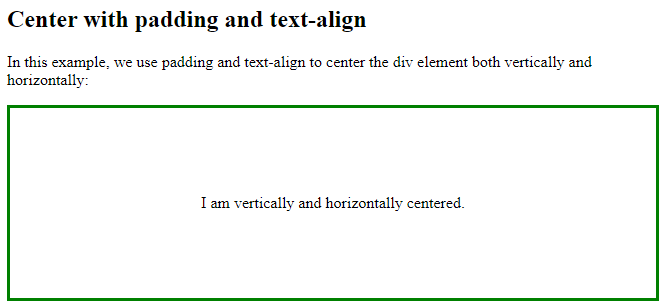
Center Vertically – Using line-height
Another trick is to use the line-height
property with a value that is equal to the height
property:
Example
<!DOCTYPE html> <html> <head> <style> .center { line-height: 200px; height: 200px; border: 3px solid green; text-align: center; } .center p { line-height: 1.5; display: inline-block; vertical-align: middle; } </style> </head> <body> <h2>Center with line-height</h2> <p>In this example, we use the line-height property with a value that is equal to the height property to center the div element:</p> <div class="center"> <p>I am vertically and horizontally centered.</p> </div> </body> </html>
Result:
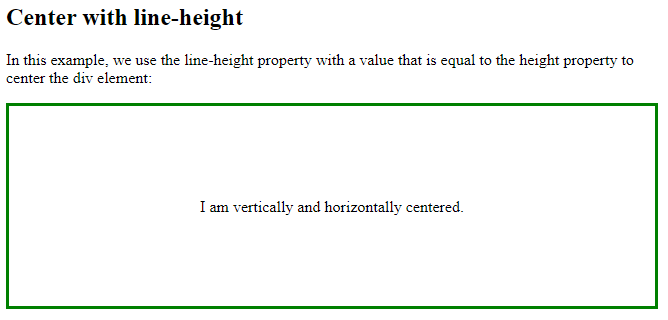
Center Vertically – Using position & transform
If padding
and line-height
are not options, another solution is to use positioning and the transform
property:
Example
<!DOCTYPE html> <html> <head> <style> .center { height: 200px; position: relative; border: 3px solid green; } .center p { margin: 0; position: absolute; top: 50%; left: 50%; -ms-transform: translate(-50%, -50%); transform: translate(-50%, -50%); } </style> </head> <body> <h2>Center with position and transform</h2> <p>In this example, we use positioning and the transform property to vertically and horizontally center the div element:</p> <div class="center"> <p>I am vertically and horizontally centered.</p> </div> </body> </html>
Result:
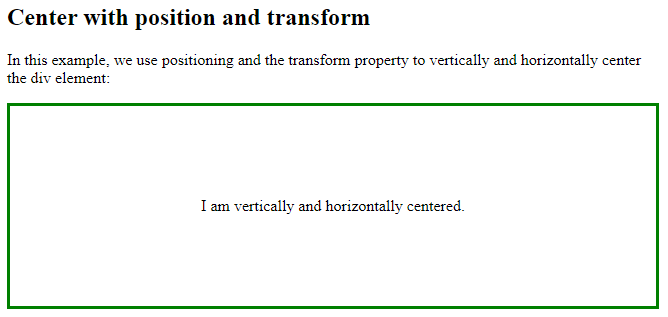
Center Vertically – Using Flexbox
You can also use flexbox to center things. Just note that flexbox is not supported in IE10 and earlier versions:
Example
<!DOCTYPE html> <html> <head> <style> .center { display: flex; justify-content: center; align-items: center; height: 200px; border: 3px solid green; } </style> </head> <body> <h2>Flexbox Centering</h2> <p>A container with both the justify-content and the align-items properties set to <em>center</em> will align the item(s) in the center (in both axis).</p> <div class="center"> <p>I am vertically and horizontally centered.</p> </div> </body> </html
Result:
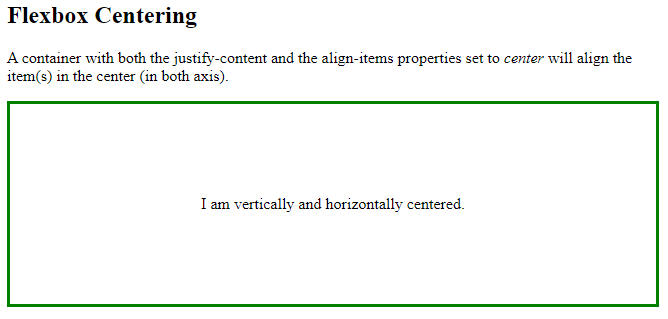