Grid Layout
The CSS Grid Layout Module offers a grid-based layout system, with rows and columns, making it easier to design web pages without having to use floats and positioning.
Browser Support
The grid properties are supported in all modern browsers.
Google Chrome | Internet Explorer | Mozilla Firefox | Safari | Opera |
---|---|---|---|---|
57.0 | 16.0 | 52.0 | 10 | 44 |
Grid Elements
A grid layout consists of a parent element, with one or more child elements.
Example
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>Grid Elements</h1> <p>A Grid Layout must have a parent element with the <em>display</em> property set to <em>grid</em> or <em>inline-grid</em>.</p> <p>Direct child element(s) of the grid container automatically becomes grid items.</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
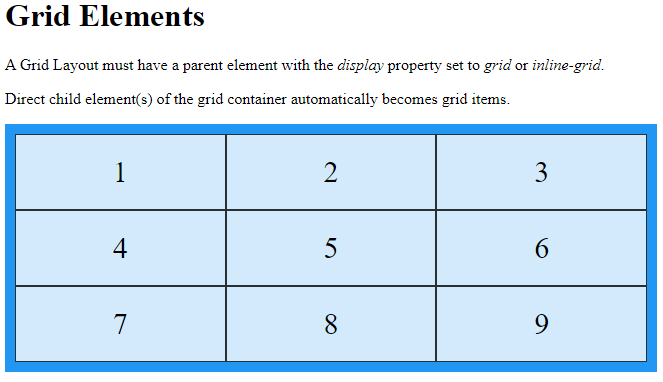
Display Property
An HTML element becomes a grid container when its display
property is set to grid
or inline-grid
.
Example
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>display: grid</h1> <p>Use display: grid; to make a block-level grid container:</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
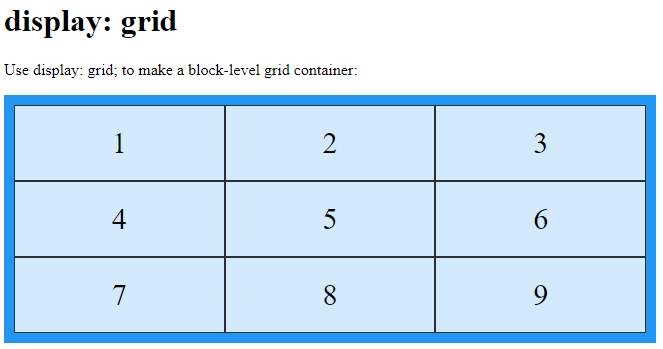
Example
<!DOCTYPE html> <html> <head> <style> .grid-container { display: inline-grid; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>display: inline-grid</h1> <p>Use display: inline-grid; to make an inline grid container:</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
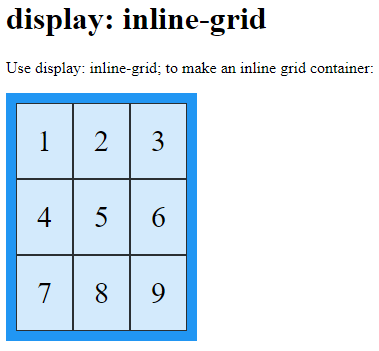
All direct children of the grid container automatically become grid items.
Grid Columns
The vertical lines of grid items are called columns.
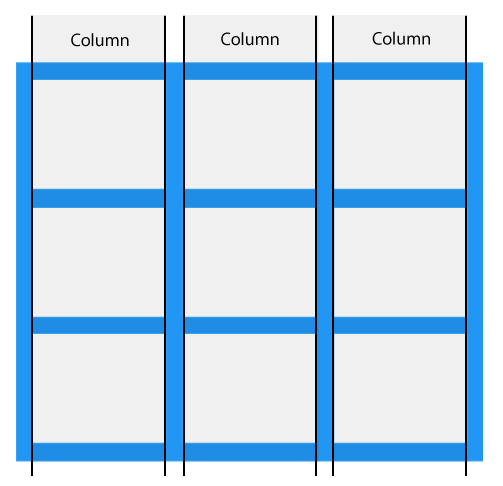
Grid Rows
The horizontal lines of grid items are called rows.
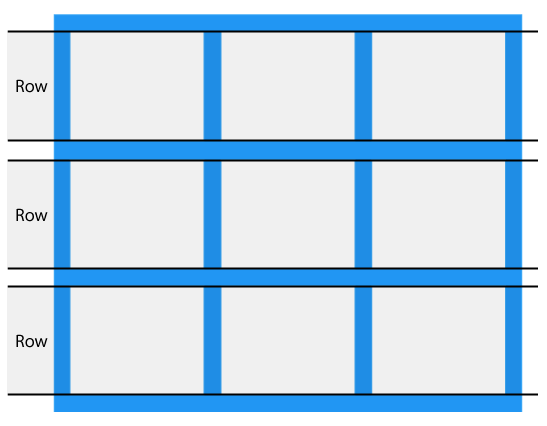
Grid Gaps
The spaces between each column/row are called gaps.
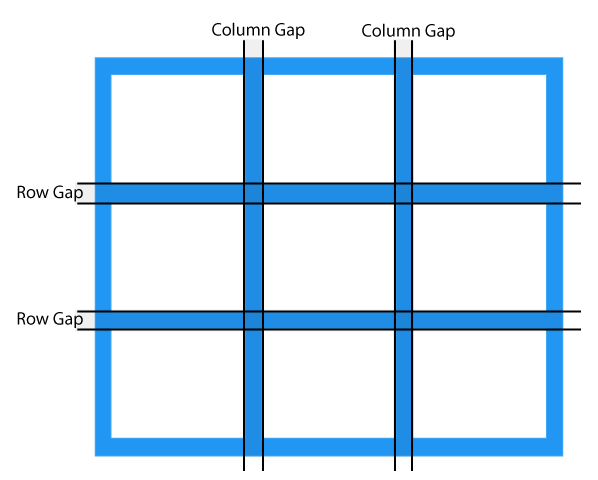
You can adjust the gap size by using one of the following properties:
column-gap
row-gap
gap
Example
The column-gap
property sets the gap between the columns:
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; column-gap: 50px; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>The column-gap Property</h1> <p>Use the <em>column-gap</em> property to adjust the space between the columns:</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
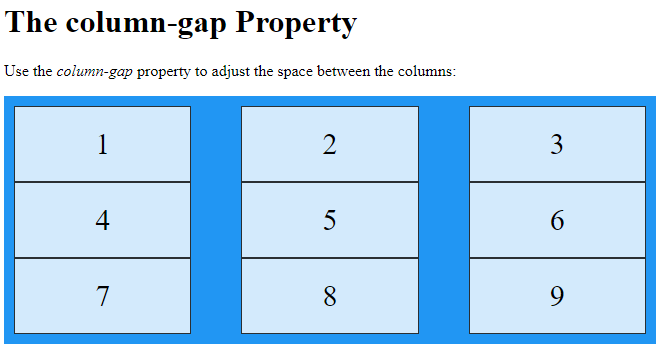
Example
The row-gap
property sets the gap between the rows:
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; row-gap: 50px; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>The row-gap Property</h1> <p>Use the <em>row-gap</em> property to adjust the space between the rows:</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
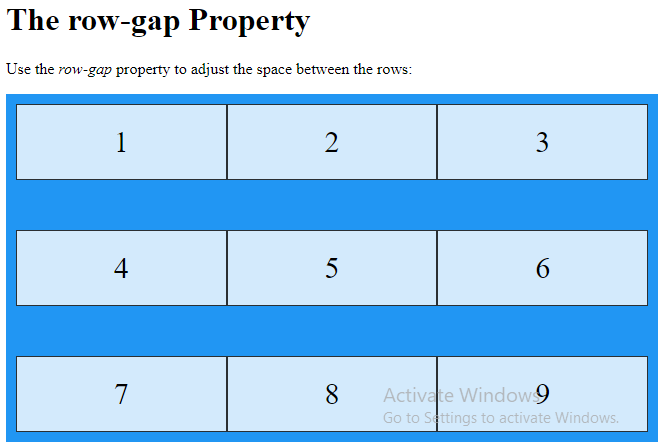
Example
The gap
property is a shorthand property for the row-gap
and the column-gap
properties:
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; gap: 50px 100px; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>The gap Property</h1> <p>Use the <em>gap</em> shorthand property to adjust the space between the columns and rows.</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
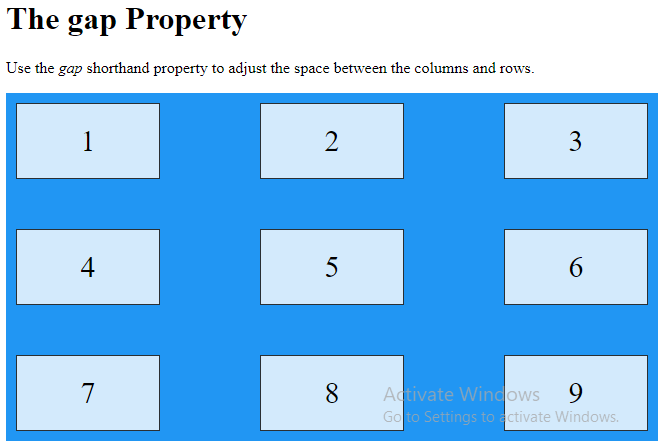
Example
The gap
property can also be used to set both the row gap and the column gap in one value:
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; gap: 50px; grid-template-columns: auto auto auto; background-color: #2196F3; padding: 10px; } .grid-item { background-color: rgba(255, 255, 255, 0.8); border: 1px solid rgba(0, 0, 0, 0.8); padding: 20px; font-size: 30px; text-align: center; } </style> </head> <body> <h1>The gap Property:</h1> <p>Use the <em>gap</em> shorthand property to adjust the space between the columns and rows:</p> <div class="grid-container"> <div class="grid-item">1</div> <div class="grid-item">2</div> <div class="grid-item">3</div> <div class="grid-item">4</div> <div class="grid-item">5</div> <div class="grid-item">6</div> <div class="grid-item">7</div> <div class="grid-item">8</div> <div class="grid-item">9</div> </div> </body> </html>
Result:
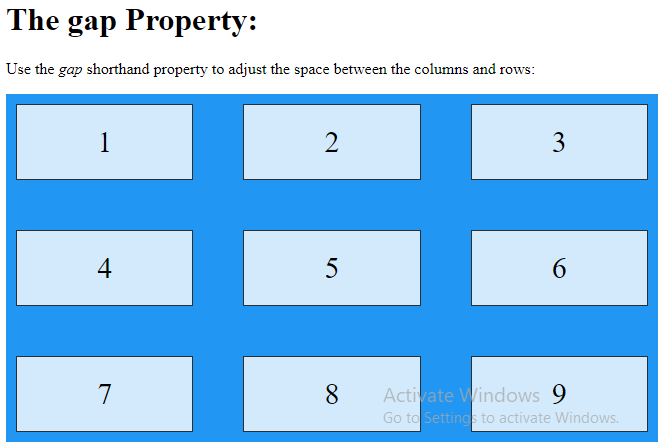
Grid Lines
The lines between columns are called column lines.
The lines between rows are called row lines.
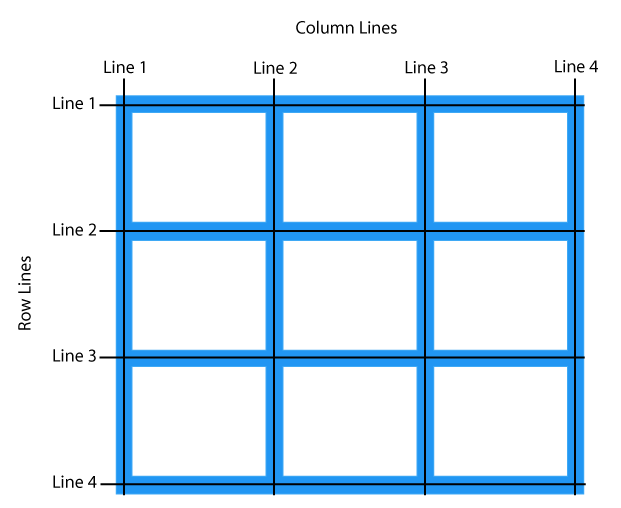
Refer to line numbers when placing a grid item in a grid container:
Example
Place a grid item at column line 1, and let it end on column line 3:
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; grid-template-columns: auto auto auto; gap: 10px; background-color: #2196F3; padding: 10px; } .grid-container > div { background-color: rgba(255, 255, 255, 0.8); text-align: center; padding: 20px 0; font-size: 30px; } .item1 { grid-column-start: 1; grid-column-end: 3; } </style> </head> <body> <h1>Grid Lines</h1> <div class="grid-container"> <div class="item1">1</div> <div class="item2">2</div> <div class="item3">3</div> <div class="item4">4</div> <div class="item5">5</div> <div class="item6">6</div> <div class="item7">7</div> <div class="item8">8</div> </div> <p>You can refer to line numbers when placing grid items.</p> </body> </html>
Result:
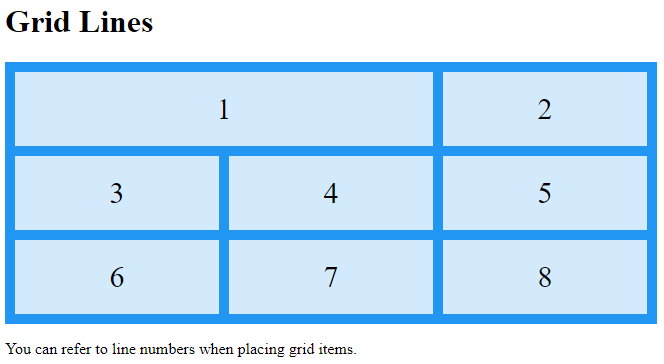
Example
Place a grid item at row line 1, and let it end on row line 3:
<!DOCTYPE html> <html> <head> <style> .grid-container { display: grid; grid-template-columns: auto auto auto; gap: 10px; background-color: #2196F3; padding: 10px; } .grid-container > div { background-color: rgba(255, 255, 255, 0.8); text-align: center; padding: 20px 0; font-size: 30px; } .item1 { grid-row-start: 1; grid-row-end: 3; } </style> </head> <body> <h1>Grid Lines</h1> <div class="grid-container"> <div class="item1">1</div> <div class="item2">2</div> <div class="item3">3</div> <div class="item4">4</div> <div class="item5">5</div> <div class="item6">6</div> <div class="item7">7</div> <div class="item8">8</div> </div> <p>You can refer to line numbers when placing grid items.</p> </body> </html>
Result:
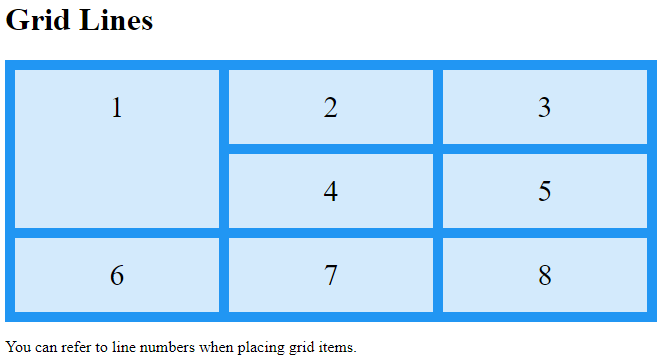