Multiple inheritance is the process of deriving a new class that inherits the attributes from two or more classes.
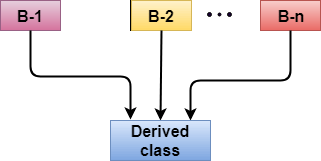
Syntax of the Derived class:
class D : visibility B-1, visibility B-2, ?
{
// Body of the class;
}
Let’s see a simple example of multiple inheritance.
#include <iostream>
using namespace std;
class A
{
protected:
int a;
public:
void get_a(int n)
{
a = n;
}
};
class B
{
protected:
int b;
public:
void get_b(int n)
{
b = n;
}
};
class C : public A,public B
{
public:
void display()
{
std::cout << “The value of a is : ” <<a<< std::endl;
std::cout << “The value of b is : ” <<b<< std::endl;
cout<<“Addition of a and b is : “<<a+b;
}
};
int main()
{
C c;
c.get_a(10);
c.get_b(20);
c.display();
return 0;
}
Output:
The value of a is : 10 The value of b is : 20 Addition of a and b is : 30
In the above example, class ‘C’ inherits two base classes ‘A’ and ‘B’ in a public mode.
Ambiquity Resolution in Inheritance
Ambiguity can be occurred in using multiple inheritance when a function with the same name occurs in more than one base class.
Let’s understand this through an example:
#include <iostream>
using namespace std;
class A {
public:
void display()
{
std::cout << “Class A” << std::endl;
}
};
class B {
public:
void display()
{
std::cout << “Class B” << std::endl;
}
};
class C : public A, public B
{
void view()
{
display();
}
};
int main()
{
C c;
c.display();
return 0;
}
Output:
error: reference to 'display' is ambiguous display();
- The above issue can be resolved by using the class resolution operator with the function. In the above example, the derived class code can be rewritten as:
class C : public A, public B
{
void view()
{
A :: display(); // Calling the display() function of class A.
B :: display(); // Calling the display() function of class B.
}
};
Ambiguity can also occur in single inheritance.
Consider the following situation:
class A
{
public:
void display()
{
cout<<?Class A?;
}
} ;
class B
{
public:
void display()
{
cout<<?Class B?;
}
} ;
In the above case, the function of the derived class overrides the method of the base class. Therefore, call to the display() function will simply call the function defined in the derived class. If we want to invoke the base class function, we can use the class resolution operator.
int main()
{
B b;
b.display(); // Calling the display() function of B class.
b.B :: display(); // Calling the display() function defined in B class.
}