Multilevel inheritance is a process of deriving a class from another derived class.
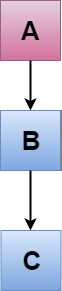
Multi Level Inheritance Example
When one class inherits another class which is further inherited by another class, it is known as multi level inheritance in C++. Inheritance is transitive so the last derived class acquires all the members of all its base classes.
Let’s see the example of multi level inheritance in C++.
#include <iostream>
using namespace std;
class Animal {
public:
void eat() {
cout<<“Eating…”<<endl;
}
};
class Dog: public Animal
{
public:
void bark(){
cout<<“Barking…”<<endl;
}
};
class BabyDog: public Dog
{
public:
void weep() {
cout<<“Weeping…”;
}
};
int main(void) {
BabyDog d1;
d1.eat();
d1.bark();
d1.weep();
return 0;
}
Output:
Eating... Barking... Weeping...