A loop statement allows us to execute a statement or group of statements multiple times. Given below is the general form of a loop statement in most of the programming languages
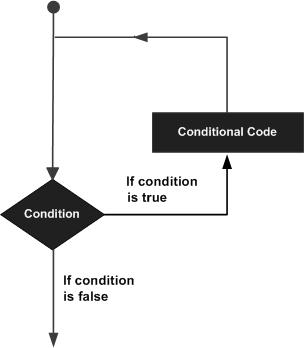
In programming, a loop is used to repeat a block of code until the specified condition is met.
C programming has three types of loops:
1. for Loop
The syntax of the for
loop is:
for (initializationStatement; testExpression; updateStatement)
{
// statements inside the body of loop
}
How for loop works?
- The initialization statement is executed only once.
- Then, the test expression is evaluated. If the test expression is evaluated to false, the
for
loop is terminated. - However, if the test expression is evaluated to true, statements inside the body of
for
loop are executed, and the update expression is updated. - Again the test expression is evaluated.
This process goes on until the test expression is false. When the test expression is false, the loop terminates.
To learn more about test expression (when the test expression is evaluated to true and false), check out relational and logical operators.
for loop Flowchart
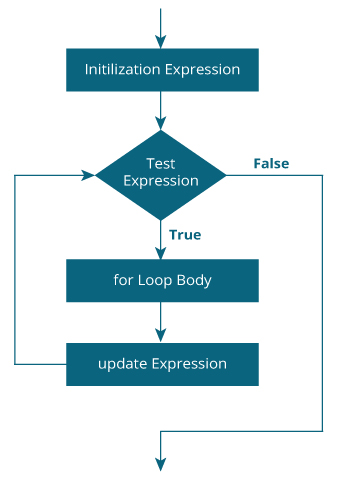
Example: for loop
// Print numbers from 1 to 10
#include <stdio.h>
int main() {
int i;
for (i = 1; i < 11; ++i)
{
printf("%d ", i);
}
return 0;
}
Output
1 2 3 4 5 6 7 8 9 10
- i is initialized to 1.
- The test expression is evaluated. Since 1 less than 11 is true, the body of for loop is executed. This will print the 1 (value of i) on the screen.
- The update statement
++i
is executed. Now, the value of i will be 2. Again, the test expression is evaluated to true, and the body of for loop is executed. This will print 2 (value of i) on the screen. - Again, the update statement
++i
is executed and the test expressioni < 11
is evaluated. This process goes on until i becomes 11. - When i becomes 11, i < 11 will be false, and the
for
loop terminates.
2. while loop
The syntax of the while
loop is:
while (testExpression)
{
// statements inside the body of the loop
}
How while loop works?
- The
while
loop evaluates the test expression inside the parenthesis()
. - If the test expression is true, statements inside the body of
while
loop are executed. Then, the test expression is evaluated again. - The process goes on until the test expression is evaluated to false.
- If the test expression is false, the loop terminates (ends).
To learn more about test expression (when the test expression is evaluated to true and false), check out relational and logical operators.
Flowchart of while loop
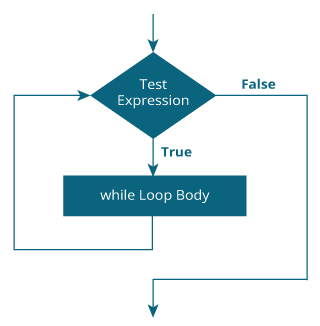
Example: while loop
// Print numbers from 1 to 5
#include <stdio.h>
int main()
{
int i = 1;
while (i <= 5)
{
printf("%d\n", i);
++i;
}
return 0;
}
Output
1 2 3 4 5
Here, we have initialized i to 1.
- When i is 1, the test expression
i <= 5
is true. Hence, the body of thewhile
loop is executed. This prints 1 on the screen and the value of i is increased to 2. - Now, i is 2, the test expression
i <= 5
is again true. The body of thewhile
loop is executed again. This prints 2 on the screen and the value ofi
is increased to 3. - This process goes on until i becomes 6. When i is 6, the test expression
i <= 5
will be false and the loop terminates.
3. do…while loop
The do..while
loop is similar to the while
loop with one important difference. The body of do...while
loop is executed at least once. Only then, the test expression is evaluated.
The syntax of the do...while
loop is:
do
{
// statements inside the body of the loop
}
while (testExpression);
How do…while loop works?
- The body of do…while loop is executed once. Only then, the test expression is evaluated.
- If the test expression is true, the body of the loop is executed again and the test expression is evaluated.
- This process goes on until the test expression becomes false.
- If the test expression is false, the loop ends.
Flowchart of do…while Loop
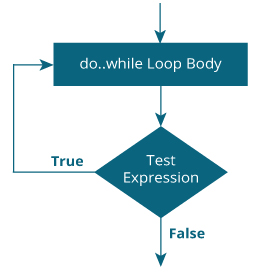
Example: do…while loop
#include<stdio.h>
#include<conio.h>
int main()
{
int num=1; //initializing the variable
do //do-while loop
{
printf("%d\n",2*num);
num++; //incrementing operation
}while(num<=10);
return 0;
}
Output
2 4 6 8 10 12 14 16 18 20
In the above example, we have printed multiplication table of 2 using a do-while loop. Let’s see how the program was able to print the series.
- First, we have initialized a variable ‘num’ with value 1. Then we have written a do-while loop.
- In a loop, we have a print function that will print the series by multiplying the value of num with 2.
- After each increment, the value of num will increase by 1, and it will be printed on the screen.
- Initially, the value of num is 1. In a body of a loop, the print function will be executed in this way: 2*num where num=1, then 2*1=2 hence the value two will be printed. This will go on until the value of num becomes 10. After that loop will be terminated and a statement that is immediately after the loop will be executed. In this case return 0.