This chapter describes the different types for the HTML <input>
element.
HTML <input> type Attribute
Here are the different input types you can use in HTML:
<input type="button">
<input type="checkbox">
<input type="color">
<input type="date">
<input type="datetime-local">
<input type="email">
<input type="file">
<input type="hidden">
<input type="image">
<input type="month">
<input type="number">
<input type="password">
<input type="radio">
<input type="range">
<input type="reset">
<input type="search">
<input type="submit">
<input type="tel">
<input type="text">
<input type="time">
<input type="url">
<input type="week">
Input Type Text
<input type="text">
defines a single-line text input field:
Example
<!DOCTYPE html> <html> <body> <h2>Text field</h2> <p>The <strong>input type="text"</strong> defines a one-line text input field:</p> <form action="/action_page.php"> <label for="fname">First name:</label><br> <input type="text" id="fname" name="fname"><br> <label for="lname">Last name:</label><br> <input type="text" id="lname" name="lname"><br><br> <input type="submit" value="Submit"> </form> <p>Note that the form itself is not visible.</p> <p>Also note that the default width of a text field is 20 characters.</p> </body> </html>
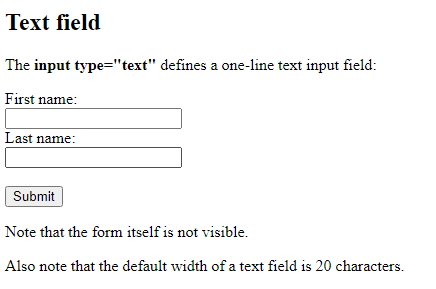
Input Type Password
<input type="password">
defines a password field:
Example
<!DOCTYPE html> <html> <body> <h2>Password field</h2> <p>The <strong>input type="password"</strong> defines a password field:</p> <form action="/action_page.php"> <label for="username">Username:</label><br> <input type="text" id="username" name="username"><br> <label for="pwd">Password:</label><br> <input type="password" id="pwd" name="pwd"><br><br> <input type="submit" value="Submit"> </form> <p>The characters in a password field are masked (shown as asterisks or circles).</p> </body> </html>
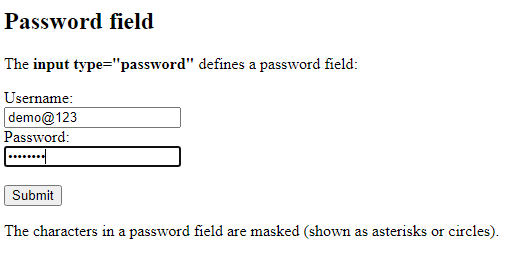
Input Type Submit
<input type="submit">
defines a button for submitting form data to a form-handler.
The form-handler is typically a server page with a script for processing input data.
The form-handler is specified in the form’s action
attribute:
Example
<!DOCTYPE html> <html> <body> <h2>Submit Button</h2> <p>The <strong>input type="submit"</strong> defines a button for submitting form data to a form-handler:</p> <form action="/action_page.php"> <label for="fname">First name:</label><br> <input type="text" id="fname" name="fname" value="John"><br> <label for="lname">Last name:</label><br> <input type="text" id="lname" name="lname" value="Doe"><br><br> <input type="submit" value="Submit"> </form> <p>If you click "Submit", the form-data will be sent to a page called "/action_page.php".</p> </body> </html>
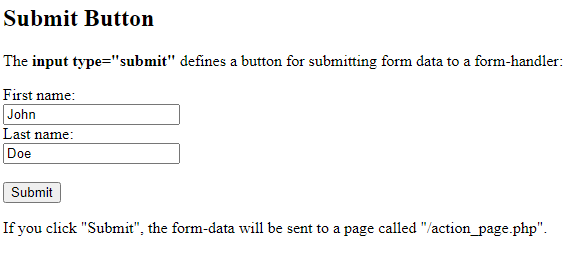
If you omit the submit button’s value attribute, the button will get a default text:
Example
<form action=”/action_page.php”>
<label for=”fname”>First name:</label><br>
<input type=”text” id=”fname” name=”fname” value=”John”><br>
<label for=”lname”>Last name:</label><br>
<input type=”text” id=”lname” name=”lname” value=”Doe”><br><br>
<input type=”submit”>
</form>
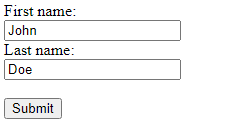
Input Type Reset
<input type="reset">
defines a reset button that will reset all form values to their default values:
Example
<!DOCTYPE html> <html> <body> <h2>Reset Button</h2> <p>The <strong>input type="reset"</strong> defines a reset button that resets all form values to their default values:</p> <form action="/action_page.php"> <label for="fname">First name:</label><br> <input type="text" id="fname" name="fname" value="John"><br> <label for="lname">Last name:</label><br> <input type="text" id="lname" name="lname" value="Doe"><br><br> <input type="submit" value="Submit"> <input type="reset"> </form> <p>If you change the input values and then click the "Reset" button, the form-data will be reset to the default values.</p> </body> </html>
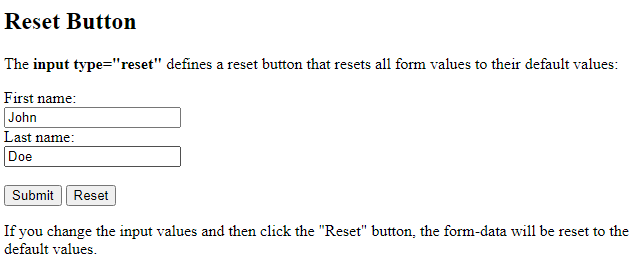
Radio Buttons
The <input type="radio">
defines a radio button.
Radio buttons let a user select ONE of a limited number of choices.
Example
A form with radio buttons:
<!DOCTYPE html> <html> <body> <h2>Radio Buttons</h2> <p>Choose your favorite Web language:</p> <form> <input type="radio" id="html" name="fav_language" value="HTML"> <label for="html">HTML</label><br> <input type="radio" id="css" name="fav_language" value="CSS"> <label for="css">CSS</label><br> <input type="radio" id="javascript" name="fav_language" value="JavaScript"> <label for="javascript">JavaScript</label> </form> </body> </html>
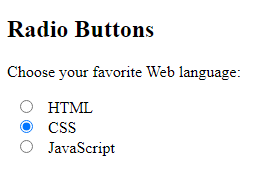
Checkboxes
The <input type="checkbox">
defines a checkbox.
Checkboxes let a user select ZERO or MORE options of a limited number of choices.
Example
A form with checkboxes:
<!DOCTYPE html> <html> <body> <h2>Checkboxes</h2> <p>The <strong>input type="checkbox"</strong> defines a checkbox:</p> <form action="/action_page.php"> <input type="checkbox" id="vehicle1" name="vehicle1" value="Bike"> <label for="vehicle1"> I have a bike</label><br> <input type="checkbox" id="vehicle2" name="vehicle2" value="Car"> <label for="vehicle2"> I have a car</label><br> <input type="checkbox" id="vehicle3" name="vehicle3" value="Boat"> <label for="vehicle3"> I have a boat</label><br><br> <input type="submit" value="Submit"> </form> </body> </html>
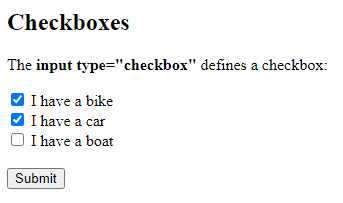
Input Type Button
<input type="button">
defines a button:
Example
<!DOCTYPE html> <html> <body> <h2>Input Button</h2> <input type="button" onclick="alert('Hello World!')" value="Click Me!"> </body> </html>
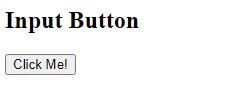
Input Type Color
The <input type="color">
is used for input fields that should contain a color.
Depending on browser support, a color picker can show up in the input field.
Example
<!DOCTYPE html> <html> <body> <h2>Show a Color Picker</h2> <p>The <strong>input type="color"</strong> is used for input fields that should contain a color.</p> <form action="/action_page.php"> <label for="favcolor">Select your favorite color:</label> <input type="color" id="favcolor" name="favcolor" value="#ff0000"> <input type="submit" value="Submit"> </form> <p><b>Note:</b> type="color" is not supported in Internet Explorer 11 or Safari 9.1 (or earlier).</p> </body> </html>
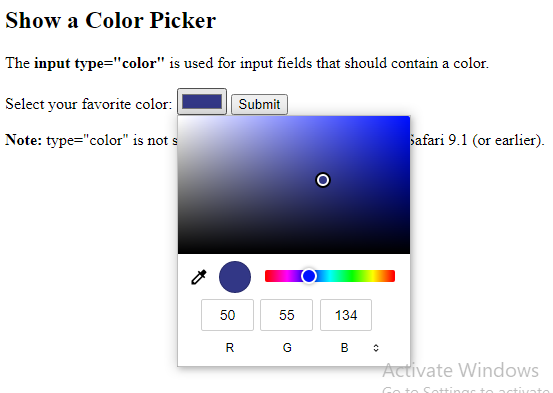
Input Type Date
The <input type="date">
is used for input fields that should contain a date.
Depending on browser support, a date picker can show up in the input field.
Example
<!DOCTYPE html> <html> <body> <h2>Date Field</h2> <p>The <strong>input type="date"</strong> is used for input fields that should contain a date.</p> <form action="/action_page.php"> <label for="birthday">Birthday:</label> <input type="date" id="birthday" name="birthday"> <input type="submit" value="Submit"> </form> <p><strong>Note:</strong> type="date" is not supported in Internet Explorer 11 or prior Safari 14.1.</p> </body> </html>
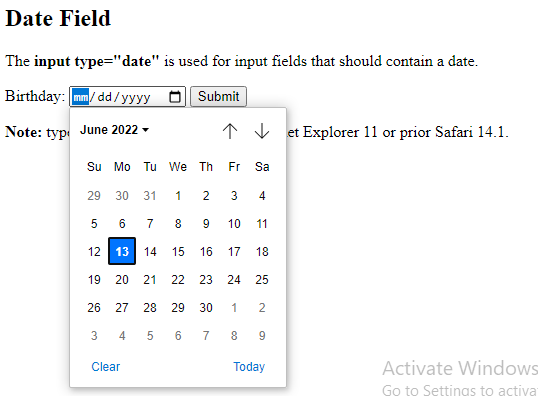
You can also use the min
and max
attributes to add restrictions to dates:
Example
<form>
<label for=”datemax”>Enter a date before 1980-01-01:</label>
<input type=”date” id=”datemax” name=”datemax” max=”1979-12-31″><br><br>
<label for=”datemin”>Enter a date after 2000-01-01:</label>
<input type=”date” id=”datemin” name=”datemin” min=”2000-01-02″>
</form>
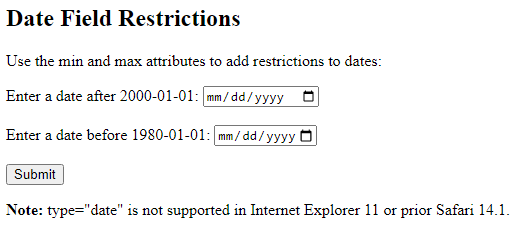
Input Type Datetime-local
The <input type="datetime-local">
specifies a date and time input field, with no time zone.
Depending on browser support, a date picker can show up in the input field.
Example
<!DOCTYPE html> <html> <body> <h2>Local Date Field</h2> <p>The <strong>input type="datetime-local"</strong> specifies a date and time input field, with no time zone.</p> <form action="/action_page.php"> <label for="birthdaytime">Birthday (date and time):</label> <input type="datetime-local" id="birthdaytime" name="birthdaytime"> <input type="submit" value="Submit"> </form> <p><strong>Note:</strong> type="datetime-local" is not supported in Internet Explorer 11 or prior Safari 14.1.</p> </body> </html>
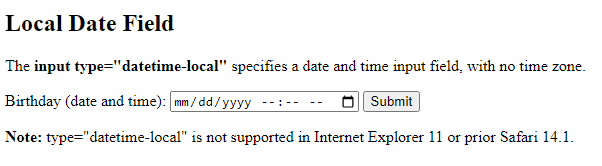
Input Type Email
The <input type="email">
is used for input fields that should contain an e-mail address.
Depending on browser support, the e-mail address can be automatically validated when submitted.
Some smartphones recognize the email type, and add “.com” to the keyboard to match email input.
Example
<!DOCTYPE html> <html> <body> <h2>Email Field</h2> <p>The <strong>input type="email"</strong> is used for input fields that should contain an e-mail address:</p> <form action="/action_page.php"> <label for="email">Enter your email:</label> <input type="email" id="email" name="email"> <input type="submit" value="Submit"> </form> </body> </html>
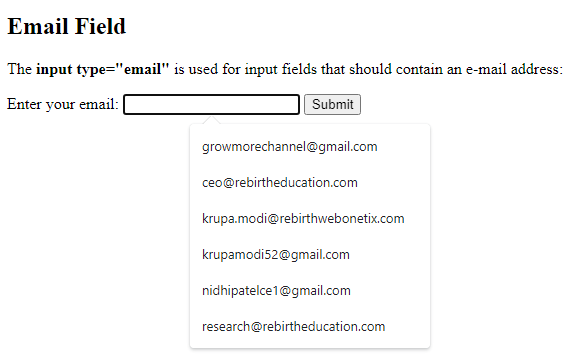
Input Type File
The <input type="file">
defines a file-select field and a “Browse” button for file uploads.
Example
<!DOCTYPE html> <html> <body> <h1>File upload</h1> <p>Show a file-select field which allows a file to be chosen for upload:</p> <form action="/action_page.php"> <label for="myfile">Select a file:</label> <input type="file" id="myfile" name="myfile"><br><br> <input type="submit" value="Submit"> </form> </body> </html>
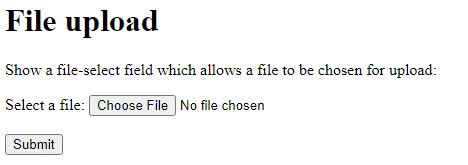
Input Type Hidden
The <input type="hidden">
defines a hidden input field (not visible to a user).
A hidden field lets web developers include data that cannot be seen or modified by users when a form is submitted.
A hidden field often stores what database record that needs to be updated when the form is submitted.
Note: While the value is not displayed to the user in the page’s content, it is visible (and can be edited) using any browser’s developer tools or “View Source” functionality. Do not use hidden inputs as a form of security!
Example
<!DOCTYPE html> <html> <body> <h1>A Hidden Field (look in source code)</h1> <form action="/action_page.php"> <label for="fname">First name:</label> <input type="text" id="fname" name="fname"><br><br> <input type="hidden" id="custId" name="custId" value="3487"> <input type="submit" value="Submit"> </form> <p><strong>Note:</strong> The hidden field is not shown to the user, but the data is sent when the form is submitted.</p> </body> </html>
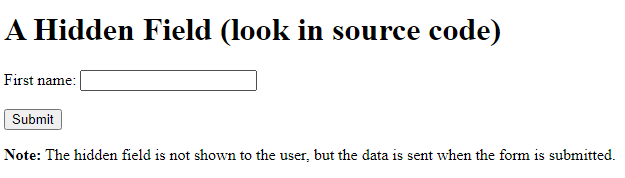
Input Type Month
The <input type="month">
allows the user to select a month and year.
Depending on browser support, a date picker can show up in the input field.
Example
<!DOCTYPE html> <html> <body> <h2>Month Field</h2> <p>The <strong>input type="month"</strong> allows the user to select a month and year.</p> <form action="/action_page.php"> <label for="bdaymonth">Birthday (month and year):</label> <input type="month" id="bdaymonth" name="bdaymonth"> <input type="submit" value="Submit"> </form> <p><strong>Note:</strong> type="month" is not supported in Firefox, Safari, or Internet Explorer 11.</p> </body> </html>
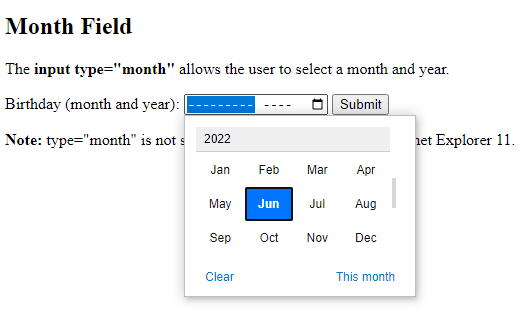
Input Type Number
The <input type="number">
defines a numeric input field.
You can also set restrictions on what numbers are accepted.
The following example displays a numeric input field, where you can enter a value from 1 to 5:
Example
<!DOCTYPE html> <html> <body> <h2>Number Field</h2> <p>The <strong>input type="number"</strong> defines a numeric input field.</p> <p>You can use the min and max attributes to add numeric restrictions in the input field:</p> <form action="/action_page.php"> <label for="quantity">Quantity (between 1 and 5):</label> <input type="number" id="quantity" name="quantity" min="1" max="5"> <input type="submit" value="Submit"> </form> </body> </html>
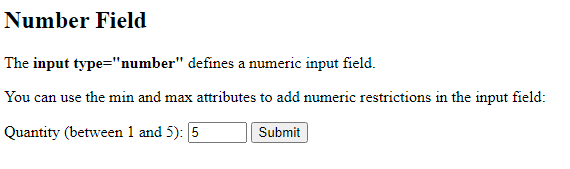
Input Restrictions
Here is a list of some common input restrictions:
Attribute | Description |
---|---|
checked | Specifies that an input field should be pre-selected when the page loads (for type=”checkbox” or type=”radio”) |
disabled | Specifies that an input field should be disabled |
max | Specifies the maximum value for an input field |
maxlength | Specifies the maximum number of character for an input field |
min | Specifies the minimum value for an input field |
pattern | Specifies a regular expression to check the input value against |
readonly | Specifies that an input field is read only (cannot be changed) |
required | Specifies that an input field is required (must be filled out) |
size | Specifies the width (in characters) of an input field |
step | Specifies the legal number intervals for an input field |
value | Specifies the default value for an input field |
You will learn more about input restrictions in the next chapter.
The following example displays a numeric input field, where you can enter a value from 0 to 100, in steps of 10. The default value is 30:
Example
<!DOCTYPE html> <html> <body> <h2>Numeric Steps</h2> <p>Depending on browser support: Fixed steps will apply in the input field.</p> <form action="/action_page.php"> <label for="quantity">Quantity:</label> <input type="number" id="quantity" name="quantity" min="0" max="100" step="10" value="30"> <input type="submit" value="Submit"> </form> </body> </html>
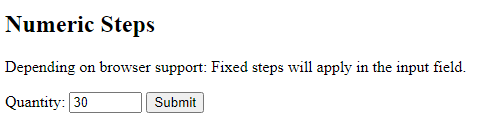
Input Type Range
The <input type="range">
defines a control for entering a number whose exact value is not important (like a slider control). Default range is 0 to 100. However, you can set restrictions on what numbers are accepted with the min
, max
, and step
attributes:
Example
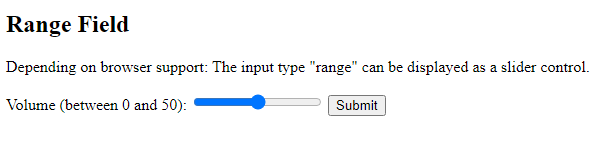
Input Type Search
The <input type="search">
is used for search fields (a search field behaves like a regular text field).
Example
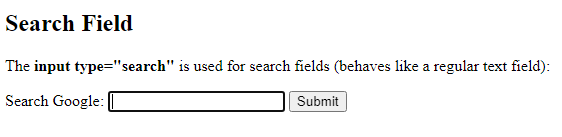
Input Type Tel
The <input type="tel">
is used for input fields that should contain a telephone number.
Example
<!DOCTYPE html> <html> <body> <h2>Telephone Field</h2> <p>The <strong>input type="tel"</strong> is used for input fields that should contain a telephone number:</p> <form action="/action_page.php"> <label for="phone">Enter a phone number:</label><br><br> <input type="tel" id="phone" name="phone" placeholder="123-45-678" pattern="[0-9]{3}-[0-9]{2}-[0-9]{3}" required><br><br> <small>Format: 123-45-678</small><br><br> <input type="submit" value="Submit"> </form> </body> </html>
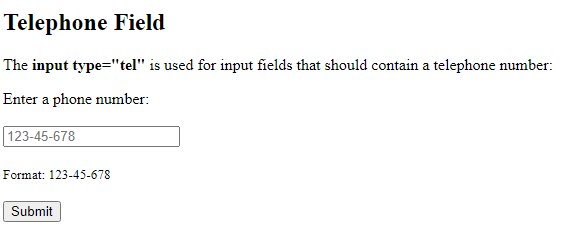
Input Type Time
The <input type="time">
allows the user to select a time (no time zone).
Depending on browser support, a time picker can show up in the input field.
Example
<!DOCTYPE html> <html> <body> <h1>Show a Time Input Control</h1> <p>The <strong>input type="time"</strong> allows the user to select a time (no time zone):</p> <p>If the browser supports it, a time picker pops up when entering the input field.</p> <form action="/action_page.php"> <label for="appt">Select a time:</label> <input type="time" id="appt" name="appt"> <input type="submit" value="Submit"> </form> <p><strong>Note:</strong> type="time" is not supported in Internet Explorer 11 or prior Safari 14.1.</p> </body> </html>
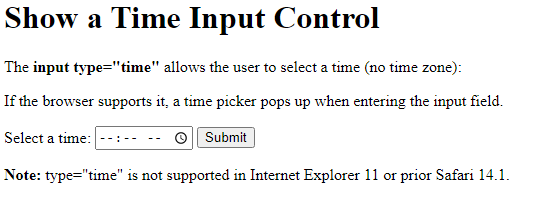
Input Type Url
The <input type="url">
is used for input fields that should contain a URL address.
Depending on browser support, the url field can be automatically validated when submitted.
Some smartphones recognize the url type, and adds “.com” to the keyboard to match url input.
Example
<!DOCTYPE html> <html> <body> <h1>Display a URL Input Field</h1> <p>The <strong>input type="url"</strong> is used for input fields that should contain a URL address:</p> <form action="/action_page.php"> <label for="homepage">Add your homepage:</label> <input type="url" id="homepage" name="homepage"> <input type="submit" value="Submit"> </form> </body> </html>
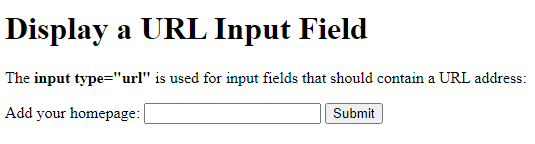
Input Type Week
The <input type="week">
allows the user to select a week and year.
Depending on browser support, a date picker can show up in the input field.
Example
<!DOCTYPE html> <html> <body> <h1>Display a Week Input Control</h1> <p>The <strong>input type="week"</strong> allows the user to select a week and year.</p> <p>If the browser supports it, a date picker pops up when entering the input field.</p> <form action="/action_page.php"> <label for="week">Select a week:</label> <input type="week" id="week" name="week"> <input type="submit" value="Submit"> </form> <p><strong>Note:</strong> type="week" is not supported in Firefox, Safari or Internet Explorer 11.</p> </body> </html>
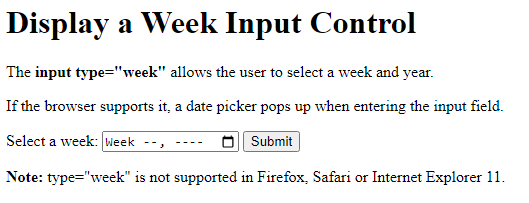